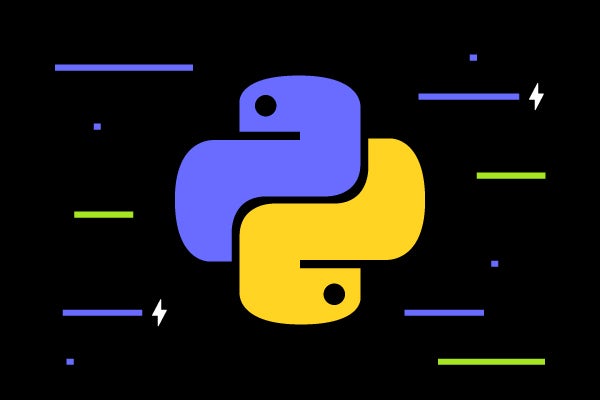
How to Debug Python in Mixed-Language Applications With SWIG and TotalView
Python is one of the most widely used programming languages. It can be used to call other high-level languages such as C, C++, and Fortran in order to provide access to high-performance routines without having to rewrite existing code.
In this article, you'll learn what SWIG is and see how to debug a mixed language C/Python example using the SWIG framework.
What Is SWIG?
Back to topSWIG — the Simplified Wrapper and Interface Generator — is an open-source software tool used to connect software written in C or C++ with scripting languages such as Perl, PHP, Python, R, Ruby, and TCL.
Debug Python With SWIG in Mixed-Language Applications
Requirements
This example uses Ubuntu 19 and Python 3.7
sudo apt-get install python-dev
sudo apt-get install python-dbg
sudo apt-get install swig
Step-by-Step
1. Start with an example.c file
/* File: example.c */
#include "example.h"
int fact(int n) {
if (n < 0){
return 0;
}
if (n == 0){
return 1;
}
else {
return n * fact(n-1);
}
}
2. Add a header file example.h
/* File: example.h */
int fact(int n);
3. Define a SWIG module example.i
/* File: example.i */
%module example
%{
#define SWIG_FILE_WITH_INIT
#include "example.h"
%}
int fact(int n);
As well as SWIG TotalView also provides support for ctypes and pybind11 extension frameworks. Other Python extension technologies will work with TotalView however additional frames will appear in the TotalView stack frame between C/C++ and Python.
4. Run swig on the SWIG module example.i to create the C and Python wrappers example_wrap.c and example.py
$swig -python example.i
5. Create a setup.py file for compiling with distutils, which comes with Python.
#!/usr/bin/env python
"""
setup.py file for SWIG example
"""
from distutils.core import setup, Extension
example_module = Extension('_example',
extra_compile_args=['-std=c99','-O'],
sources=['example_wrap.c', 'example.c'],
)
setup (name = 'example',
version = '0.1',
author = "Perforce",
description = """Simple swig example""",
ext_modules = [example_module],
py_modules = ["example"],
)
6. Build the Python module.
$python3-dbg setup.py build_ext -–inplace
7. Test the Python module.

8. Write a Python test wrapper test_fact.py
# Test program for fact
import example as ex
result = ex.fact(4)
print (result)
9. Start TotalView debugger to debug the C/Python code.
$totalview -args python3-dbg test_fact.py
10. Set a pending breakpoint on the fact function.
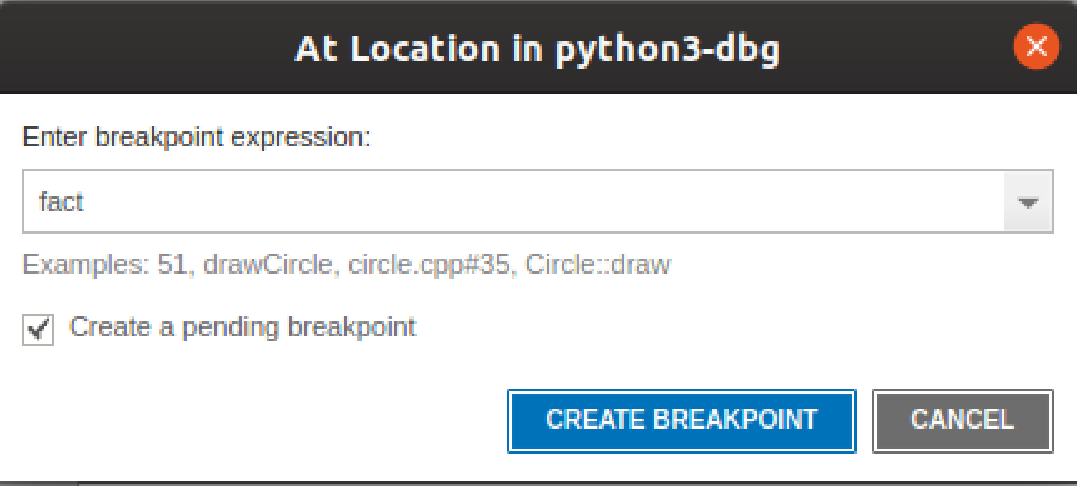
11. TotalView shows the C code.
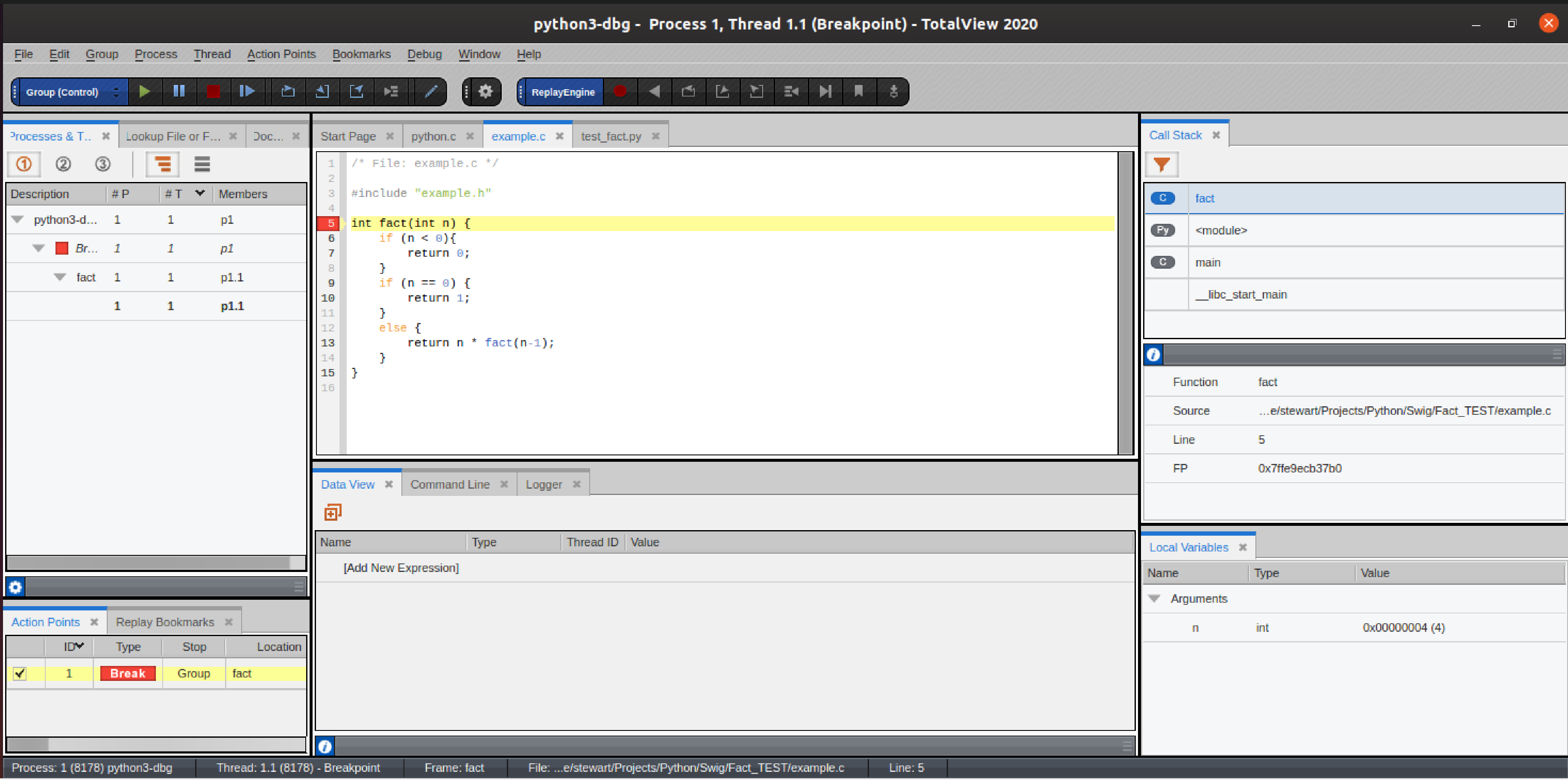
12. TotalView shows the Python code.
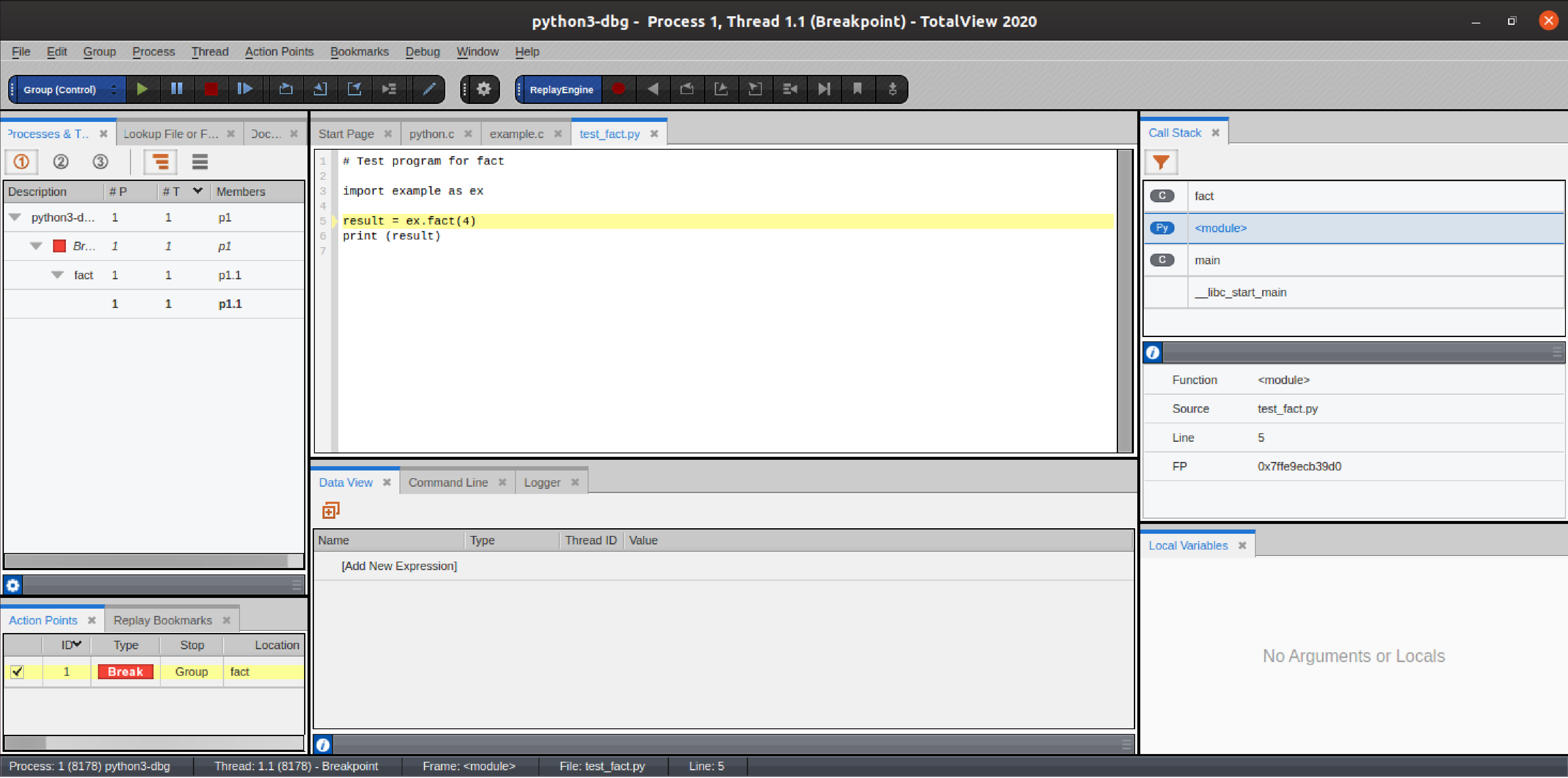
Next Steps for SWIG, Python, and TotalView
This step by step guide showed you how to debug Python in multi-language applications using SWIG and TotalView. TotalView provides a very easy workflow for establishing your Python and C++ / C debugging sessions.
Test TotalView and it's multi-language debugging capabilities with your application today.
Additional Resources
- Debugging Python and C/C++ Mixed-Language Applications (2:42 Video Tutorial)
- Python Debugging Support - TotalView User Guide
Acknowledgments
The example used in this article is from http://www.swig.org/Doc4.0/Python.html#Python_nn9
Back to top